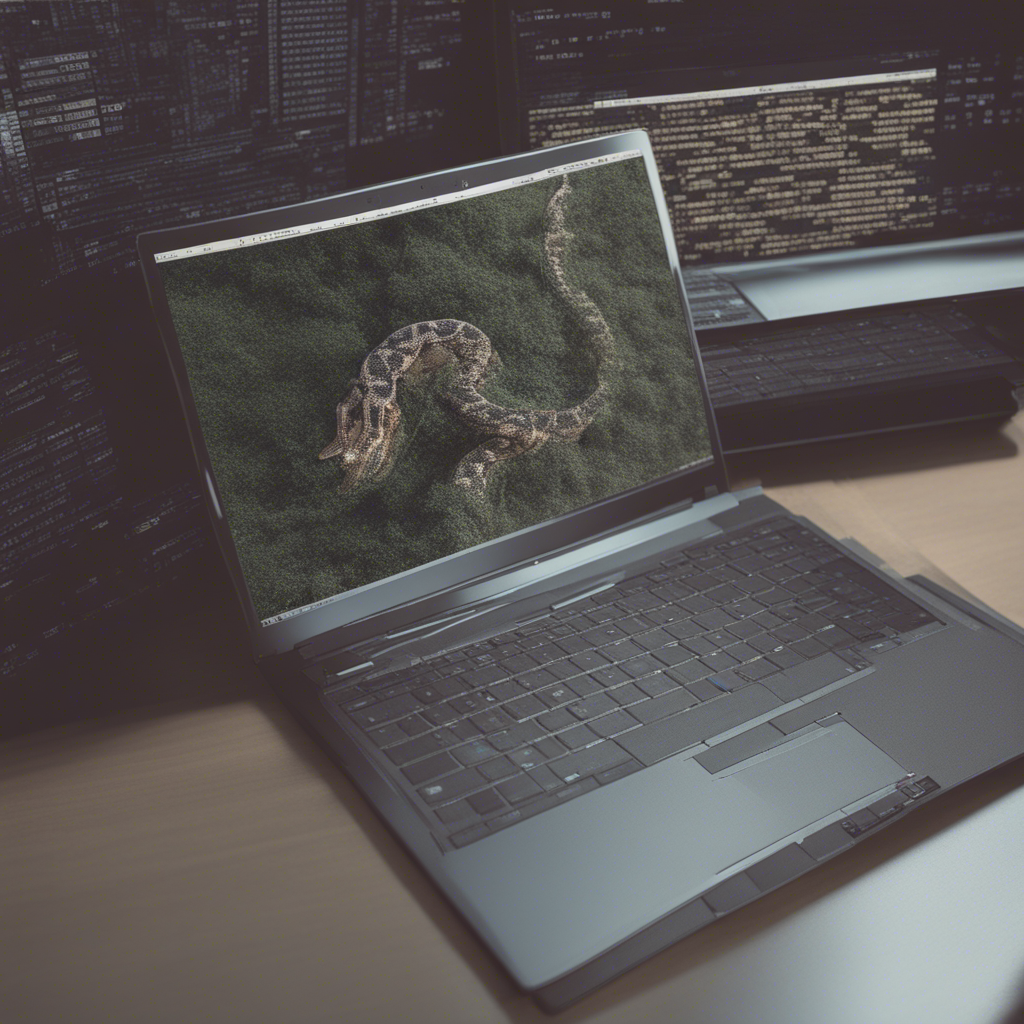
Master Python: Step by Step Tutorial for Beginners
Are you new to programming and looking to learn Python? Look no further! This comprehensive step-by-step tutorial will guide you through the basics of Python programming, equipping you with the foundational knowledge to become a proficient Python developer. Whether you’re a student, a professional looking to switch careers, or simply eager to learn a new skill, this tutorial is designed to help you master Python.
Why Python?
Python is one of the most popular programming languages in the world, known for its simplicity, versatility, and readability. It has gained immense popularity due to its wide range of applications, including web development, data analysis, machine learning, and automation. Python’s intuitive syntax and extensive libraries make it an excellent choice for beginners and experienced developers alike.
Setting up Python Development Environment
Before diving into learning Python, you need to set up your development environment. Here’s a simple step-by-step guide:
-
Download and Install Python: Visit the official Python website (python.org) and download the latest version of Python. Choose the appropriate installer for your operating system (Windows, macOS, or Linux) and follow the installation instructions. Make sure to check the option to add Python to your system’s PATH.
-
Choose a Code Editor: While Python comes with a built-in IDLE, you may find it more convenient to use a dedicated code editor. Popular choices include Visual Studio Code, PyCharm, and Atom. Pick the one that suits your preferences and install it on your system.
-
Verify Python Installation: Open your terminal or command prompt and type
python --version
. If you see the Python version installed on your system, you’re good to go!
Python Basics
Now that your development environment is all set up, let’s dive into the basics of Python programming. We’ll cover the essential concepts and syntax that form the foundation of the language.
1. Variables and Data Types
In Python, you can store values in variables. Variables have different data types depending on the type of value they hold. Some commonly used data types in Python include:
- Integers: Represent whole numbers, such as 5 or -3.
- Floats: Represent decimal numbers, such as 3.14 or -2.5.
- Strings: Represent sequences of characters, enclosed in single quotes (’’) or double quotes ("").
- Booleans: Represent the truth values True or False.
# Example of variable assignment
x = 5
y = 3.14
name = "John Doe"
is_student = True
2. Operators
Python supports a wide range of operators for performing different operations on variables and values. Some commonly used operators include:
- Arithmetic Operators: Addition (+), Subtraction (-), Multiplication (*), Division (/), Modulo (%), Exponentiation (**).
- Comparison Operators: Equal to (==), Not equal to (!=), Greater than (>), Less than (<), Greater than or equal to (>=), Less than or equal to (<=).
- Logical Operators: And (and), Or (or), Not (not).
# Example of arithmetic operations
addition_result = 5 + 3
subtraction_result = 7 - 2
division_result = 10 / 2
modulo_result = 15 % 4
exponentiation_result = 2 ** 3
3. Control Flow
Control flow statements allow you to control the execution order of statements in your code. Common control flow statements in Python include:
- Conditional Statements: If, elif, else.
- Looping Statements: For, while.
# Example of conditional statement
age = 25
if age >= 18:
print("You are eligible to vote!")
else:
print("You are not eligible to vote.")
# Example of looping statement
numbers = [1, 2, 3, 4, 5]
for number in numbers:
print(number)
while condition:
# Perform some action
pass
4. Functions and Modules
Functions allow you to encapsulate reusable pieces of code, making your programs more modular and organized. Python also offers a wide range of built-in and third-party modules to extend the functionality of your programs. Some commonly used functions and modules include:
- print(): Used to output text or variables to the console.
- range(): Used to generate a sequence of numbers.
- math: A built-in module for mathematical operations.
- random: A built-in module for generating random numbers.
# Example of function and module usage
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
import math
circle_area = math.pi * radius ** 2
Going Further with Python
Congratulations! You have now gained a solid understanding of the basics of Python programming. But this is just the tip of the iceberg. Python offers numerous advanced features and libraries that can further enhance your programming journey. Here are some topics you may want to explore next:
- Object-Oriented Programming in Python
- Working with Files and Directories
- Data Manipulation with NumPy and Pandas
- Web Development with Django or Flask
- Machine Learning with Scikit-learn or TensorFlow
Take your learning to the next level by exploring these topics and building real-world projects. Remember, practice and hands-on experience are the keys to mastering Python and becoming a proficient developer.
Conclusion
In this step-by-step tutorial, we covered the basics of Python programming, starting from setting up your development environment to understanding variables, data types, operators, control flow, functions, and modules. Python’s simplicity and versatility make it an excellent choice for beginners, and its vast ecosystem of libraries makes it suitable for a wide range of applications.
Now, armed with this foundational knowledge, you can continue your Python journey and explore the advanced features and libraries that will empower you to solve complex problems, build exciting projects, and thrive in the world of programming.
Happy coding!
Note: This tutorial is inspired by the Python official documentation and various reputable Python resources.