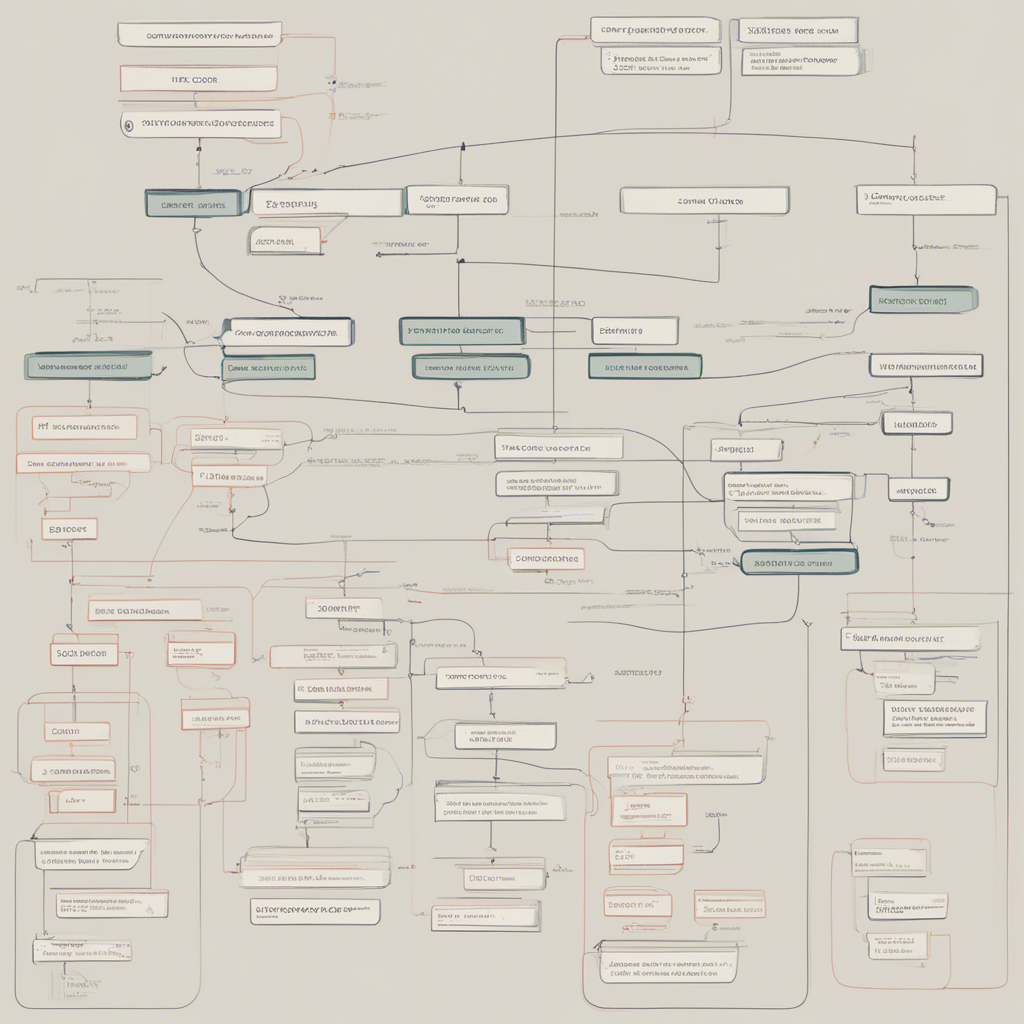
Mastering Git Best Practices for Developers
Git is a powerful and widely used version control system essential for modern software development. It allows developers to track and manage changes in code efficiently, collaborate seamlessly with team members, and ensure code quality and project stability. However, to fully leverage the capabilities of Git, developers need to follow best practices that promote efficient workflow, effective collaboration, and reliable code management.
In this comprehensive guide, we will explore and discuss the best practices and techniques that will help developers master Git and optimize their development processes. We will cover everything from setting up a Git repository to handling branches, merging changes, resolving conflicts, and more.
Table of Contents
- Introduction to Git
- Setting Up a Git Repository
- Creating and Managing Branches
- Committing Changes
- Collaborating with Team Members
- Merging Changes
- Resolving Conflicts
- Reverting Changes
- Tagging and Versioning
- Git Configurations and Customizations
- Advanced Git Techniques
- Conclusion
Before we dive into each section, let’s briefly introduce Git and highlight its importance in modern software development.
Introduction to Git
Git, developed by Linus Torvalds in 2005, is a distributed version control system primarily used in software development. It allows developers to track changes to their codebase and manage multiple versions of their project efficiently. Git achieves this by creating a local repository on each developer’s machine, allowing for offline work and distributed collaboration among team members.
Git offers several advantages over traditional version control systems, such as Subversion or CVS. It allows for branching and merging of code effortlessly, enabling developers to work on multiple features concurrently without disrupting others. It also provides detailed history and commit information, facilitating efficient bug tracking, code review, and auditing.
Setting Up a Git Repository
To start using Git, developers need to set up a repository, which will serve as the central hub for their codebase. This repository can be created locally or hosted on a remote server, such as GitHub, GitLab, or Bitbucket.
To create a local repository, navigate to the desired directory using the terminal or command line interface and run the following command:
$ git init
To create a remote repository hosted on a service like GitHub, follow the instructions provided by the platform. Once the repository is set up, developers can clone it to their local machines using the following command:
$ git clone <repository-url>
Creating and Managing Branches
Branching is one of Git’s most powerful features, allowing developers to create isolated environments to work on specific features or bug fixes. By using branches, developers can work independently without affecting the main codebase until they are ready to merge their changes.
To create a new branch, use the following command:
$ git branch <branch-name>
To switch to the newly created branch, use:
$ git checkout <branch-name>
To list all branches and see the currently active one:
$ git branch
When working on a branch, developers can commit changes and push them to the remote repository without affecting other branches or the main codebase. This promotes parallel development and allows for efficient collaboration.
Committing Changes
Committing changes is essential in Git to track and save progress on a project. A commit is like a snapshot of the codebase at a particular point in time. Each commit includes a unique identifier, author information, commit message, timestamps, and the specific changes made.
To stage changes for committing, use the following command:
$ git add .
This command stages all modified and new files in the current directory recursively. Alternatively, developers can specify the specific files to stage.
To commit the changes, execute the following command:
$ git commit -m "Commit message"
It’s important to write meaningful and descriptive commit messages that provide context about the changes made. This helps team members understand the purpose of the commit without having to inspect the code changes manually.
Collaborating with Team Members
Git enables seamless collaboration among team members. To collaborate effectively, developers can push their local changes to the remote repository for other team members to review, merge, and test.
To push changes to the remote repository, use:
$ git push
By default, this command pushes changes to the branch of the same name as the one checked out locally. Alternatively, developers can specify the branch they want to push to explicitly.
To pull changes made by other team members, use:
$ git pull
This command fetches remote changes and merges them with the local codebase. If conflicts arise, we will discuss how to resolve them later in this guide.
It is important to communicate and coordinate effectively with team members, using tools like issue tracking systems, project management platforms, or communication channels like Slack or Microsoft Teams.
Merging Changes
Once development work on a branch is complete and tested, developers can merge their changes into the main codebase. Merging incorporates the changes made on one branch into another branch, typically the main branch or the branch from which the feature branch was created.
To merge changes from one branch into another, use:
$ git merge <source-branch>
If the merge can be performed automatically without conflicts, Git will apply the changes and create a new commit with a merge message. However, if conflicts occur during the merge, developers need to manually resolve them before proceeding.
Resolving Conflicts
Conflicts arise when Git cannot automatically merge changes made on different branches due to conflicting modifications to the same file or code block. Resolving conflicts requires careful consideration and manual intervention.
To resolve conflicts, developers need to open and edit the conflicting files, marked with conflict markers. The markers highlight the conflicting regions and provide the conflicting changes from both branches. Developers must analyze the changes, decide on the correct version, and remove the markers to resolve the conflict.
After resolving conflicts, developers need to stage the resolved files and create a new commit to finalize the merge process. It is good practice to review the changes before committing to ensure the conflicts are resolved correctly.
Reverting Changes
Sometimes, it becomes necessary to undo or revert changes made in previous commits. Git provides various approaches to revert changes, depending on the desired outcome.
To revert the changes made in a specific commit, use:
$ git revert <commit-hash>
This command creates a new commit that undoes the changes made in the specified commit. It is a safe way to undo changes without modifying the commit history.
To reset the repository to a previous commit and discard all subsequent changes, use:
$ git reset <commit-hash>
This command discards commits and moves the branch pointer to the specified commit. Be cautious when using this command, as it permanently removes commits from the branch history.
Tagging and Versioning
Tagging provides a way to mark specific points in history and create meaningful snapshots, typically used for versioning or release management. Tags can be lightweight or annotated, providing additional metadata and context.
To create a lightweight tag, use:
$ git tag <tag-name>
To create an annotated tag with additional information like a message or author, use:
$ git tag -a <tag-name> -m "Tag message"
To list all tags, use:
```bash
$ git tag
Tags are useful for referencing specific points in the codebase, creating releases, or marking important milestones.
Git Configurations and Customizations
Git allows developers to configure various settings to customize the behavior of the version control system. These configurations impact everything from user information and commit messages to merge strategies.
To view and update global Git configurations, use the following commands:
$ git config --global --list
$ git config --global <configuration-name> <configuration-value>
Developers can also customize repository-specific configurations using similar commands, but without the --global
flag.
Git configurations provide flexibility and control over the Git workflow, allowing developers to tailor Git to their specific preferences and requirements.
Advanced Git Techniques
Mastering Git involves learning and utilizing advanced techniques that can further enhance development processes and efficiency. Some of these techniques include:
- Git Hooks: These are scripts executed by Git automatically at specific events, allowing developers to enforce custom checks or perform actions such as code linting, running tests, or triggering deployment processes.
- Git rebase: This technique allows developers to modify the commit history by moving, combining, or removing commits. It can be powerful but also delicate, requiring caution to avoid unintended consequences.
- Git submodules: Git submodules enable developers to include other Git repositories within a repository. This is useful for managing dependencies or including shared codebases in a project.
- Git stash: This feature allows developers to save and temporarily hide changes to the working directory, allowing them to switch branches or work on other tasks without committing incomplete changes.
Conclusion
Mastering Git is essential for developers aiming to streamline their workflow, collaborate effectively, and manage code changes efficiently. In this comprehensive guide, we have explored the best practices and techniques that can help developers optimize their Git usage.
By setting up repositories correctly, managing branches and commits effectively, collaborating with team members seamlessly, and resolving conflicts and reverting changes when necessary, developers can harness Git’s full potential and enhance their development capabilities.
Remember, Git is a widely adopted and continuously evolving tool with a vibrant community. Continuously learning and staying up-to-date with new features and best practices is crucial to mastering Git and becoming a proficient software developer.